Hello, we will make a sqlite connection with python.
Our first example is to create a very simple table, after save some data and show all records on screen.
Now let’s move on to the process steps, respectively
import to sqlite3 library
Note: This library comes internally with python 3 by default. But if it doesn’t come, run this command
pip install pysqlite3
import sqlite3
I will create a physical database file and get the cursor object.
conn = sqlite3.connect("contact.db") cursor = conn.cursor()
Define a table creator method and make the table scheme here.
def create_table(): cursor.execute("CREATE TABLE IF NOT EXISTS person_info(" "id integer PRIMARY KEY AUTOINCREMENT," "name text," "number text," "city text," "alias text)")
Let’s run the method and close the connections and look at the result
create_table() cursor.close() conn.close()
We see that a file named contact.db has occurred in the directory where we run our .py file.

Let’s open this file with free sqlite editor. DB Browser for SQLite
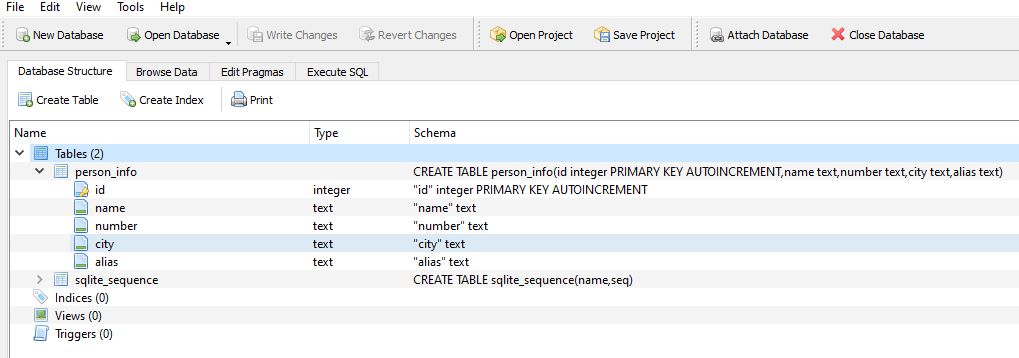
Its ok. we have reached our goal. We made a simple introduction. In my next post, I will further develop this small project. What will happen? I will rewrite it exactly with object oriented logic. I will dynamically add data entry and search feature.